Preferences allow you to store basic information about your .NET MAUI app, such as your user name, to speed up your interactions. Let’s take a look!
Storing simple information on your device, such as remembering your email or username, can help speed up and facilitate user interactions in your app. Logging in with a username saved users time, which directly impacts their experience with our products.
Consider another scenario where you need to show an onboarding screen every time a user logs in from a new device. You can also store information about whether they have already been onboarded on this device. All of this is possible in .NET MAUI using Preferences.
Preferences are used to store this information on your device, providing an easy-to-access alternative to simple data without the need for a database.
Let’s get started!
To utilize Preferences, you must use the IPreferences interface. All accessible properties can be accessed using Preferences.Default, which is in the Microsoft.Maui.Storage namespace.
Preference Structure
Preferences consist of two important elements:
🔹 core: This is a unique identifier for the preference. Essentially, it’s a name we’ll use to reference the value stored in that preference. string By value. For example: user_email
.
🔹value: This is the value you want to store in relation to the key. For example, an image represents a value. leo@gmail.com
It will be saved in your preferences user_email
. You can use this key to search and modify as needed. The following sections discuss the types of data we allow.
Allowed storage types
It’s important to remember that you can only use a limited set of data types to store values in a Preference. These are:
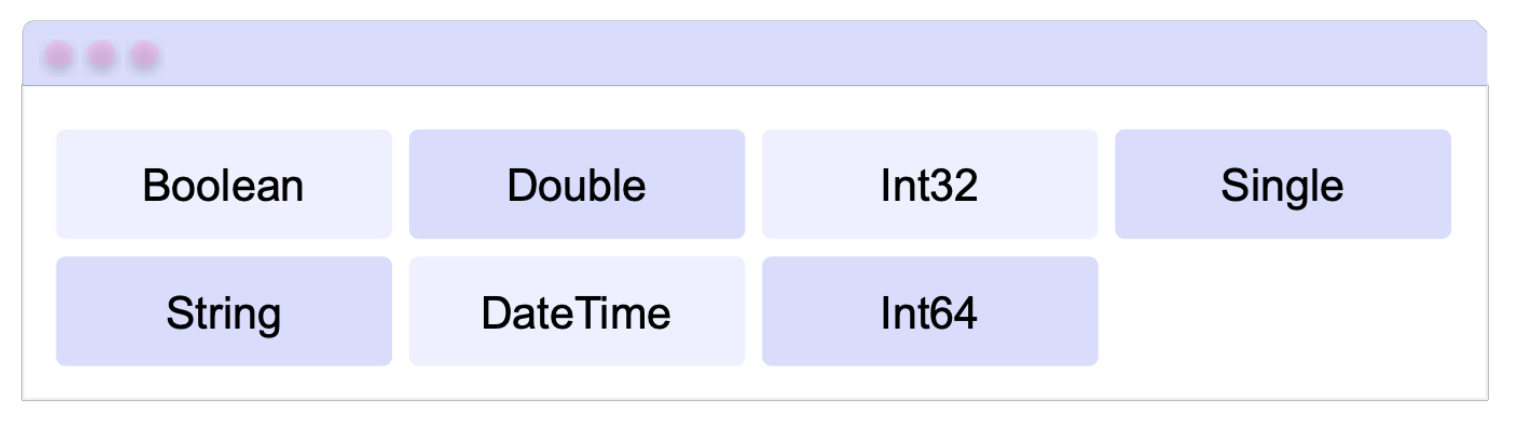
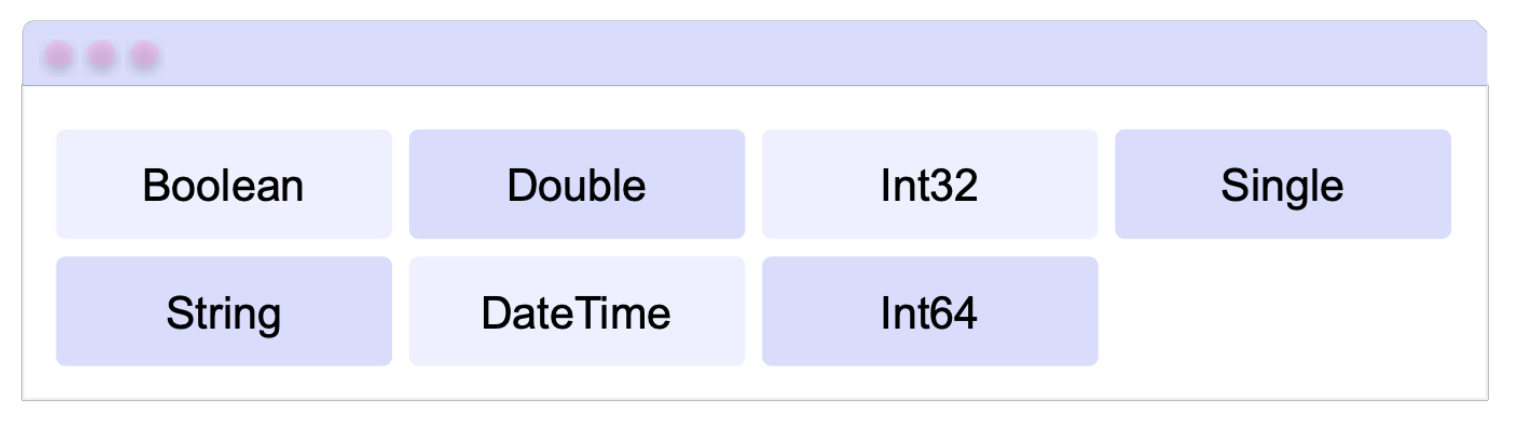
Values stored as DateTime are stored in 64-bit binary (long integer) format using two methods defined in the DateTime class.
ToBinary to encode a DateTime value
🔹 FromBinary to decode the value
Review the documentation for these methods to understand how to handle special cases that may arise when using DateTime.
Manipulating Preferences
Now that we’ve established the necessary background context, let’s take a look at the save preferences.
Setting up your environment
To set preference values, use: Set
Method. As discussed previously, it requires preferred key and value as parameters.
In code it looks like this:
Preferences.Default.Set("user_name", "Leo");
Preferences.Default.Set("user_has_email",true);
Preferences.Default.Set("user_email", "leo@gmail.com");
Get preference
To retrieve a key value, use: Get
Method. This method requires two parameters.
- that Key name You want to search
- no way Default value to be returned If the key does not exist.
The line of code should look like this:
string userName = Preferences.Default.Get("user_name", "Unknown");
bool hasEmail = Preferences.Default.Get("user_has_email", true);
bool userEmail = Preferences.Default.Get("user_email", "Unknown");
Check if there are any preferences
You can also check if a key exists using: ContainsKey
Method. Just pass the name of the key you want to verify. This method returns a boolean value.
bool hasEmail = Preferences.Default.ContainsKey("user_has_email");
Remove key
There are two ways to remove a key.
🔹 Remove specific keys: Use it Remove
Select a method and enter the name of the key you want to delete.
Preferences.Default.Remove("user_name");
🔹 Remove all existing keys: Easy to use Clear
method.
Preferences.Default.Clear();
Share the key
Initially, saved preferences are only visible within the application in which they were created. However, if necessary, you can create shared preferences for use by other extensions or applications.
The Set, Get, Remove, and Clear methods have the following optional parameters: sharedName
(defaults to null) Specifies the container where the preferences are stored.
Here’s an example of how to do this:
Preferences.Default.Set("user_email", "[leo@gmail.com](mailto:leo@gmail.com)", "shared_user_email"),
⚠️ It is important to understand the specific implementation details of each platform, as shared preferences are implemented with specific behaviors.
Platform Differences
LocalSettings limits preference key names to a maximum of 255 characters. Each preference value can be up to 8KB in size, and each composite configuration can be up to 64KB in size.
✍️ Things to remember
If you uninstall the app, your preferences will be removed. However, this issue does not occur on Android 6.0 (API level 23) and higher if the Auto Backup feature is enabled. This feature is enabled by default.
🚫 Limitations
The default setting should be used for small or simple values. It may affect performance when used on large amounts of data. If you need more storage, I personally recommend using a database.
References
This document is based on the official documentation.