Learn about four .NET MAUI Community Toolkit actions that can make cross-platform app development easy!
๐ง The .NET MAUI Community Toolkit is a curated collection of reusable components carefully developed by the community. It includes a variety of elements such as animations, transformers, actions, and more, all designed to accelerate app development. Plus, the power of .NET MAUI ensures seamless compatibility across iOS, Android, macOS, and WinUI.
In this article, you’ll learn about four fantastic moves from the .NET MAUI Community Toolkit that can power up your applications. ๐ fat? Now you are ready to use! All you need is to understand how to implement it.
The actions we will look at are:
- email validator
- masked
- icon color tone
- Vigor
Each of these actions in the .NET MAUI Community Toolkit is designed to provide specific improvements to your app.
But first, letโs pause for a moment. What exactly is action? ๐ค
Actions allow you to add specific functionality to view elements. These modular features not only improve reusability but also streamline the unit testing process.
โ๏ธ If you want to know more about the operation, please check the official documentation article.
Initial settings
To implement the behavior correctly, make sure the .NET MAUI Community Toolkit is properly configured in your app. Setup is simple as described in the steps below.
- installation: First install the toolkit by adding the Community.Toolkit.Maui NuGet package.
- Setting in MauiProgram.cs: After adding the NuGet package, navigate to MauiProgram.cs. right below
UseMauiApp<App>()
addition:
.UseMauiCommunityToolkit()
- Add namespace: Include the Toolkit namespace in your page.
xmlns:toolkit="[http://schemas.microsoft.com/dotnet/2022/maui/toolkit](http://schemas.microsoft.com/dotnet/2022/maui/toolkit)"
1. EmailValidator operation
This action detects whether the provided text is a valid email address using a regular expression. As a result, developers can easily provide visual feedback to users regarding the validity of their emails. For example, imagine something where the font color changes to red for invalid emails and green for valid emails.
When paired with an InputView (e.g. Entry or Editor), this action automatically sets the keyboard to Keyboard.Email unless a different default keyboard is specified.
Let’s start with a hands-on code example.
script
We are designing an item where the font size is set to 25 and the text is displayed in dark red when the email is invalid. Conversely, a valid email would have a font size of 30 and green text.
Create a style
For each scenario, we’ll define a style that encapsulates the desired visual properties.
โ Invalid email:
<Style x:Key="InvalidEmailStyle" TargetType="Entry">
<Setter Property="FontSize" Value="25"/>
<Setter Property="TextColor" Value="DarkRed"/>
</Style>
โ Valid email:
<Style x:Key="ValidaEmailStyle" TargetType="Entry">
<Setter Property="FontSize" Value="30"/>
<Setter Property="TextColor" Value="Green"/>
</Style>
validation properties
EmailValidator boasts several properties, the most notable of which are:
โช wrong style and valid style โ Receive style By value. This is the style displayed to the user when the email is invalid or valid respectively.
โช flag โ receive validation flag By value. This enumeration defines how validation is managed. The values โโof this enumeration are:
- ValidateOnAttaching: When an action is connected, it triggers validation.
- ValidateOnFocusing: The control validates input as soon as it gains focus.
- ValidateOnUnfocusing: Validates input when the control loses focus.
- ValidateOnValueChanged: Validation starts whenever the input value changes.
- ForceMakeValidWhenFocused: Forces the input to appear valid when focused, regardless of its actual validity.
- doesn’t exist: Automatic validation is not applied.
Add action
now Email validation behavior Keep the previously discussed properties in mind and add them to your entry.
<Entry>
<Entry.Behaviors>
<toolkit:EmailValidationBehavior
InvalidStyle="{StaticResource InvalidEmailStyle}"
ValidStyle="{StaticResource ValidaEmailStyle}"
Flags="ValidateOnValueChanged" />
</Entry.Behaviors>
</Entry>
The result should be similar to this:
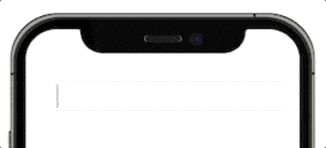
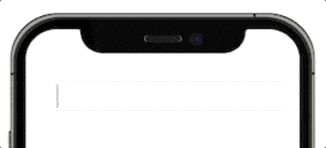
masked behavior
that much masked behavior Users can apply an input mask to specific data items, ensuring consistency by only accepting values โโthat match the given mask. This is especially useful for standardized data formats such as credit card numbers, phone numbers, etc.
Let’s start with a hands-on code example.
script
We are setting up a mask for Dominican Republic phone numbers. Given the following sample numbers: (800) 222-4434The mask format is: (XXX) XXX-XXXX.
masked properties
Two properties that help define a mask are:
โช mask โ Receives a string as value. Specifies a mask pattern that the input value must conform to. essential. There are two ways to set this mask.
- Enter the string directly in the desired format. yes:
XXX XXX XXXX
. - Introduces non-replaceable characters into the format. for example,
(XXX) XXX XXXX
. Here the parentheses do not change, but instead appear as you type the format. To utilize this feature effectively, you must also use the UnmaskedCharacter property.
โช Unmasked character โ Indicates the character within the mask attribute that will be replaced by user input. Characters not specified in this property will still be displayed to the user. Referring to the previous example, the value of this property is: X
Make sure parentheses are not replaced.
Add action
now masked action Keep the previously discussed properties in mind and add them to your entry.
<Entry Keyboard="Numeric" Placeholder="(XXX) XXX XXXX">
<Entry.Behaviors>
<toolkit:MaskedBehavior Mask="(XXX) XXX XXXX" UnmaskedCharacter="X" />
</Entry.Behaviors>
</Entry>
โ๏ธ Added placeholder properties to give users clearer guidance on the expected format.
The result should be:
3. IconTintColor operation
This action activates image tinting. This will prove invaluable when customizing your app for light and dark modes. Instead of requiring separate icons for each mode, you can use a single icon and adjust the tint color as per your requirements.
Let’s start with a hands-on code example.
script
Let’s apply a red tint to the icon. However, in other examples, this icon will turn orange when the device is set to dark mode, and green when in light mode.
avatar
This behavior has a single property: Hue color, which determines the hue of the icon tint. For example, to get a red icon, follow the steps below:
โ๏ธ Keep in mind that the starting color of the icon is blue.
<Image Source="heart" HeightRequest="150" WidthRequest="150">
<Image.Behaviors>
<toolkit:IconTintColorBehavior TintColor="Red" />
</Image.Behaviors>
</Image>
Now let’s play Appearance Mode.
<Image Source="heart" HeightRequest="150" WidthRequest="150">
<Image.Behaviors>
<toolkit:IconTintColorBehavior TintColor="{AppThemeBinding Dark=Orange, Light=green}" />
</Image.Behaviors>
</Image>
โ๏ธ Are you interested in mastering the user interface for different appearance modes? Take a look at the article Managing light mode and dark mode using .NET MAUI.
4. Animation behavior
This behavior is designed to smoothly animate the associated VisualElement. In default mode, we use a TapGestureRecognizer to start the animation, but this trigger activates when it detects that the user taps or clicks on a VisualElement, ensuring responsive and dynamic interaction.
Let’s start with a hands-on code example.
script
Imagine an image on a screen. When you click or tap, the image will fade smoothly.
Add action
now animation action In our images:
<Image Source="dotnet_bot.png">
<Image.Behaviors>
<toolkit:AnimationBehavior>
<toolkit:AnimationBehavior.AnimationType>
<toolkit:FadeAnimation />
</toolkit:AnimationBehavior.AnimationType>
</toolkit:AnimationBehavior>
</Image.Behaviors>
</Image>
The result should be:
โ๏ธ Curious about more animation types in .NET MAUI? Take a look at the article Basic Animated Navigation in .NET MAUI.
conclusion
๐ And there it is! With these simple steps, we have successfully implemented the previously described behavior.
We hope you found this list of useful actions in the .NET MAUI Community Toolkit helpful. I encourage you to implement this into your daily life and continue to explore others! ๐๐
Let’s meet again next time! ๐โโ๏ธ
References
This article is based on the official documentation:
Use component libraries in line with the release flow of .NET MAUI. Try Telerik UI for .NET MAUI for free.