Welcome to the Python Error Handling MCQ Quiz! Error handling is an essential aspect of programming, and Python provides powerful mechanisms for managing exceptions and errors that may occur during program execution. This quiz aims to test your understanding of various concepts related to error handling in Python, including: try
and except
Blocks, raising and catching exceptions, use finally
blocks, and more. Each question is multiple choice with one correct answer. Take your time and read each question carefully and choose the option that best suits you. Let’s get started and explore Python error handling together!
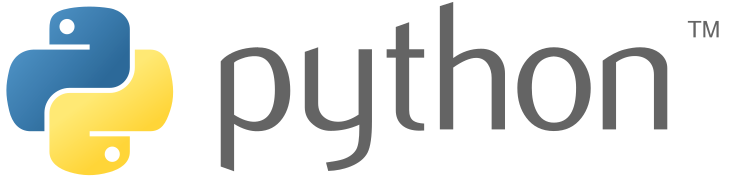
Q1. What is the purpose of a try block in Python error handling?
a) To define a block of code where an exception may occur:
b) To catch and handle exceptions that occur within a block:
c) To check if the code runs without errors:
d) To terminate the program when an exception occurs:
answer: all
explanation: A try block defines a block of code in which an exception may occur.
Q2. What keywords are used to catch exceptions in Python?
trial
b) catching
c) excluded
d) handle
answer: Seed
explanation: The Except keyword is used to catch and handle exceptions in Python.
Q3. What happens when an error occurs while running a Python program?
a) error
B) Exception
c) defect
d) bug
answer: rain
explanation: In Python, errors during execution are displayed as exceptions.
Q4. Which of the following is not a standard Python exception?
a) key error
b) ValueException
c) IndexError
d) type error
answer: rain
explanation: ValueException is not a standard Python exception. Must be a ValueError.
Q5. How can I handle multiple exceptions in a single exclude block?
a) Use commas to separate exceptions.
b) Use nesting without blocks
c) Use the Except keyword only once.
d) It is not possible to handle multiple exceptions in a single exception block.
answer: all
explanation: You can use commas to separate multiple exceptions in a single exclusion block.
Q6. What Python keywords are used to manually raise exceptions?
a) Throw
b) raise
c) Exceptions
d) trigger
answer: rain
explanation: The raise keyword is used to manually raise exceptions in Python.
Q7. What does a finally block guarantee in Python error handling?
a) Ensures that the code within it is always executed, regardless of whether an exception occurs or not.
b) Ensures that the program terminates when an exception occurs.
c) Causes the program to skip executing code when an exception occurs.
d) When an exception occurs, only the code within the finally block is executed.
answer: all
explanation: A finally block ensures that the code within it always runs, regardless of whether an exception occurs.
Q8. Which of the following statements about the else block in Python error handling is correct?
a) Executed when an exception occurs.
b) It is executed if no exception is raised in the try block.
c) Handle any exceptions that occur in the try block.
d) Executed instead of a finally block.
answer: rain
explanation: Python executes the else block if no exception occurs in the try block.
Q9. What is the purpose of Assert statement in Python?
a) To handle the exception
b) To exit the program
c) To check if the condition is true
d) To raise an exception
answer: Seed
explanation: The Assert statement checks if a condition is true in Python. If the condition is false, an AssertionError is raised.
Q10.Which of the following is not a commonly used method of the Exception class in Python?
a) __str__()
b) __init__()
c) __Cause__()
d) __repr__()
answer: Seed
explanation: __cause__() is not a method of the Exception class. Used to determine the cause of an exception.
Q11. Which of the following is true about Python’s Except clause?
a) Required in Try-Exception blocks.
b) It catches all exceptions by default.
c) Must be placed before the try block.
d) You can specify the type of exception you want to catch.
answer: d
explanation: The Except clause can specify the type of exception to catch.
Q12. What is the output of the following code?
try:
x = 10 / 0
except ZeroDivisionError:
print("Division by zero")
finally:
print("Finally block")
a) Divide by 0
last blocked
b) finally block
c) divide by zero
d) ZeroDivisionError
answer: all
explanation: The code raises a ZeroDivisionError, catches it, prints “Division by zero”, and finally blocks printing “Finally block”.
Q13. Which of the following keywords is used to handle exception blocks in Python?
hand
b) structure
c) excluded
d) catching
answer: Seed
explanation: Except is used to handle exception blocks in Python.
Q14. What does the following Python code do?
try:
# Some code that may raise an exception
except:
pass
a) An exception is thrown.
b) Catch and ignore all exceptions.
c) Close the program.
d) Handle exceptions gracefully.
answer: rain
explanation: This code catches all exceptions and ignores them due to the pass statement.
Q15. What happens if an exception occurs in the finally block itself?
a) Exceptions are caught by Except blocks.
b) The program ends.
c) The exception propagates up the call stack.
d) Exceptions are ignored.
answer: Seed
explanation: If an exception occurs in the finally block itself, it is propagated up the call stack.
Q16. Which of the following is not a common built-in exception in Python?
a) key error
b) FileNotFoundError
c) IndexError
d) syntax error
answer: d
explanation: SyntaxError is a general syntax error, but is not a built-in exception class.
Q17. What is the purpose of the sys.exc_info() function in Python?
a) An exception is thrown.
b) Returns information about the exception currently being handled.
c) Close the program.
d) Print a trace of the exception.
answer: rain
explanation: sys.exc_info() returns a tuple of information about the current exception being handled in Python.
Q18. What keyword is used to rethrow the last exception caught in Python?
a) Throw again
b) rethrow_last
c) raise_last
d) raise
answer: d
explanation: In Python, developers use the raise keyword to re-raise the last caught exception.
Q19. What is the output of the following code?
try:
raise Exception("An error occurred")
except Exception as e:
print(e)
finally:
print("Finally block")
a) An error occurred
last blocked
b) finally block
c) An error occurred.
d) Exception
answer: all
explanation: The code throws an exception, catches it, prints an error message, and then executes a finally block.
Q20. Which of the following is true about exception handling in Python?
a) An exception handler can catch exceptions raised by the function it calls.
b) Exception handlers cannot catch exceptions thrown by the functions they call.
c) Exception handlers only catch exceptions that occur in the same block.
d) Exception handlers can only catch exceptions of the same type.
answer: all
explanation: Exception handlers can catch exceptions raised by functions they call.
Q21. What is the purpose of the backtracking module in Python?
a) Error messages can be customized.
b) Print detailed information about the exception.
c) Handling exceptions in multi-threaded applications.
d) Raise an exception based on user-defined conditions.
answer: rain
explanation: The traceback module provides the ability to print detailed information about the exception.
Q22. Which of the following is a correct description of assertion statements in Python?
a) Used to handle exceptions.
b) If the condition is true, terminate the program.
c) If the condition is false, an exception is raised.
d) Similar to a Try-Exception block.
answer: Seed
explanation: If the condition is false, the Assertion statement raises AssertionError.
Q23. How can I create a custom exception class in Python?
a) By inheriting from the Exception class
b) using the throw keyword
c) Using the custom_Exception keyword:
d) by defining a function with the exception name
answer: all
explanation: You can create a custom exception class by inheriting from the Exception class.
Q24. What does the sys.exit() function do in Python?
a) Raise an exception.
b) Close the program.
c) Print a message to the console.
d) Exception handling
answer: rain
explanation: sys.exit() terminates the program.
Q25. Which of the following statements about the else block in Python error handling is correct?
a) Executed when an exception occurs.
b) Handle any exceptions that occur in the try block.
c) Executes only when an exception occurs.
d) It is executed if no exception occurs in the try block.
answer: d
explanation: If no exception occurs in the try block, Python executes the else block.
Q26. What is the purpose of the raise statement in Python?
a) To catch the exception
b) To ignore the exception
c) To re-raise the exception
d) To exit the program
answer: Seed
explanation: In Python, developers use the raise statement to re-raise an exception.
Q27. Which of the following is not a standard Python exception?
a) syntax error
b) ZeroDivisionError
c) Overflow error
d) Runtime error
answer: Seed
explanation: OverflowError is a standard Python exception, but it is not as common as the other exceptions listed.
Q28. What is the purpose of a try-out-else block in Python?
a) To catch the exception and execute replacement code:
b) To run code that should always be run:
c) To handle the exception and run the code if no exception occurs:
d) To terminate the program when an exception occurs:
answer: Seed
explanation: In Python, developers use try-out-else blocks to handle exceptions and execute code if no exceptions occur.
Q29. What keywords are used to define custom exception classes in Python?
a) catch
b) raise
c) class
d) Exception
answer: Seed
explanation: In Python, developers use the class keyword to define custom exception classes.
Q30. What is the purpose of a finally block in Python error handling?
a) To handle the exception
b) To raise an exception
c) To ensure that certain code always runs
d) To exit the program
answer: Seed
explanation: A finally block ensures that certain code will be executed regardless of whether an exception occurs.
Q31. Which of the following exceptions occurs when you try to access an index in a list that does not exist?
a) value error
b) key error
c) IndexError
d) type error
answer: Seed
explanation: An IndexError is raised if you try to access an out-of-bounds index in a list-like sequence.
Q32. Can a Try-Exception block have multiple Except blocks?
a) No, only one is allowed, excluding blocks.
b) Yes, but only if you are handling other types of exceptions.
c) Yes, regardless of the type of exception you are handling.
d) Yes, but only if they are nested within each other.
answer: rain
explanation: In Python, it is considered good practice to order multiple exclusion blocks from most specific to least specific. This is because Python checks those blocks in order and executes the first block that matches.
Q33. Which of the following is correct about the ordering of Except blocks in Try-Exception syntax?
a) The order of block exclusions does not matter.
b) The most specific exception handler should come first.
c) The most common exception handler should come first.
d) If the order of the exclude blocks is incorrect, Python raises a SyntaxError.
answer: rain
explanation: In Python, it is a good idea to sort multiple exclusion blocks from most specific to least specific. This is because Python checks the blocks in order and executes the first block that matches.
Congratulations on completing the Python Error Handling MCQ Quiz! We hope this quiz will help you strengthen your understanding of Python error handling concepts and techniques. Managing exceptions effectively is important for writing robust and reliable Python code. By mastering error handling, you can ensure that your program handles unexpected situations appropriately and provides meaningful feedback to users. To become a proficient Python developer, continue practicing and exploring Python’s error handling mechanisms. If you have any questions or want to delve deeper into a specific topic, don’t hesitate to continue your learning journey. Happy coding!
You can also sign up for a free Python course right now!
Read more articles related to MCQs in Python.