introduction
Detecting gender in face images is one of the many fascinating applications of computer vision. In this project, we will combine OpenCV for face location and Roboflow API for gender classification to create a device that identifies, verifies, and predicts gender of a face. We will use Python, specifically Google Colab, to input and run this code. This straightforward explanation provides an easy-to-follow walkthrough of the code, clearly explaining each step so that you can understand and apply it to your venture.
Learning Objectives
- Learn how to implement face detection using OpenCV’s Haar Cascade.
- Learn how to integrate the Roboflow API for gender classification.
- Explore how to process and manipulate images in Python.
- We use Matplotlib to visualize the detection results.
- We develop practical technologies that combine AI and computer vision that can be applied in the real world.
This article was published as part of: Data Science Blogthon.
How to detect gender using OpenCV and Roboflow in Python?
Let’s learn how to implement OpenCV and Roboflow in Python for gender detection.
Step 1: Import the library and upload the image
The first step is to create the important libraries. We use OpenCV to prepare the images, NumPy to process the clusters, and Matplotlib to visualize the results. We also uploaded the images containing the faces we want to analyze.
from google.colab import files
import cv2
import numpy as np
from matplotlib import pyplot as plt
from inference_sdk import InferenceHTTPClient
# Upload image
uploaded = files.upload()
# Load the image
for filename in uploaded.keys():
img_path = filename
In Google Colab, the files.upload() operation allows a client to transfer records, such as photos, from a neighboring machine to the Colab environment. Upon upload, the photo is stored in a word reference named transfer, which compares the key to the record name. A for loop is then used to extract the file path for further processing. To handle the image processing task, we use OpenCV to detect faces and draw bounding boxes around them. At the same time, we use Matplotlib to visualize the results, including displaying the images and cropped faces.
Step 2: Loading the Haar Cascade model for face detection
Next, we stack the Haar Cascade demo from OpenCV, which is pre-trained to identify faces. The model scans the image, finds patterns that resemble human faces, and returns their coordinates.
# Load the Haar Cascade model for face detection
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
A commonly used strategy for object detection. Identify edges, textures, and patterns associated with an object (in this case, a face). OpenCV provides a pre-trained face detection model that is loaded using `CascadeClassifier`.
Step 3: Detect faces in the image
We stack the transmitted photos and convert them to grayscale, which makes a difference in the progress towards location accuracy. We then use a face detector to find faces in the images.
# Load the image and convert to grayscale
img = cv2.imread(img_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Detect faces in the image
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
- Image loading and conversion:
- Stack the transmitted images using cv2.imread().
- Convert the image to grayscale using cv2.cvtColor() to reduce complexity and improve search capabilities.
- Face Detection:
- Find faces in grayscale images using detectMultiScale().
- This feature resizes the image and examines facial patterns in different areas.
- Parameters like scaleFactor and minNeighbors adjust the detection sensitivity and accuracy.
Step 4: Setting up the gender detection API
Now that we have detected the faces, we will initialize the Roboflow API using InferenceHTTPClient to predict the gender of each detected face.
# Initialize InferenceHTTPClient for gender detection
CLIENT = InferenceHTTPClient(
api_url="https://detect.roboflow.com",
api_key="USE_YOUR_API"
)
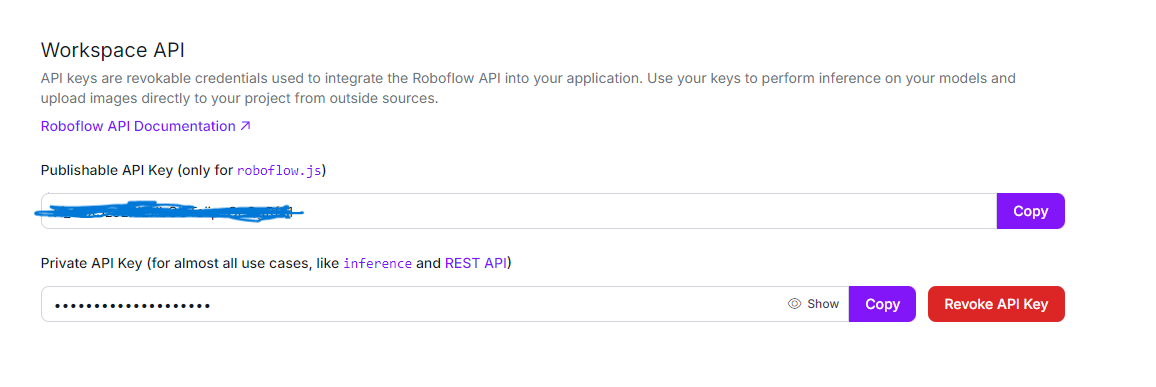
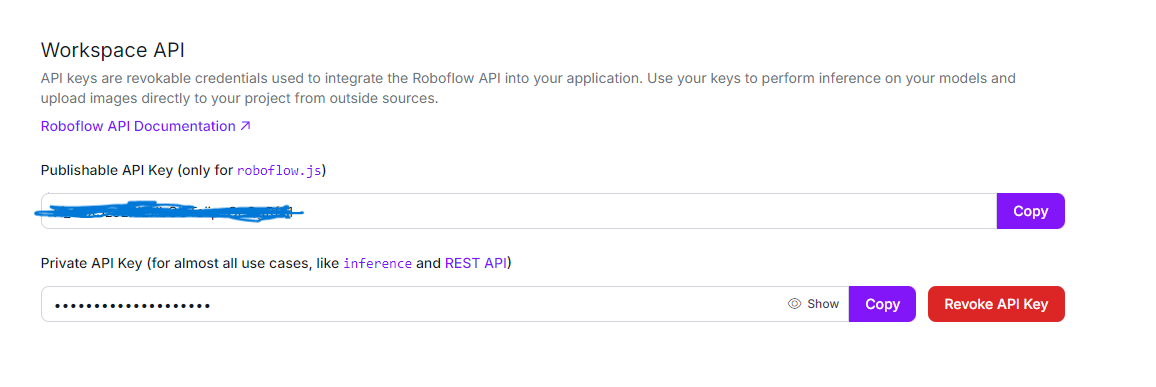
InferenceHTTPClient simplifies interaction with Roboflow’s pre-trained models by configuring the client with a Roboflow API URL and API key. This setup allows you to send requests to a gender detection model hosted on Roboflow. The API key acts as a unique identifier for authentication, allowing secure access and utilization of the Roboflow API.
Step 5: Process each detected face
We iterate over each detected face, draw a rectangle around it, and then crop the face image for further processing. Each cropped face image is temporarily stored and sent to the Roboflow API, where we use the gender-detection-qiyyg/2 model to predict the gender.
The gender-detection-qiyyg/2 model is a pre-trained deep learning model optimized to classify gender as male or female based on facial features. It provides predictions along with a confidence score indicating how confident the model is about the classification. The model is trained on a powerful dataset, allowing it to make accurate predictions across a wide range of face images. These predictions are returned by the API and used to label each face with the identified gender and confidence level.
# Initialize face count
face_count = 0
# List to store cropped face images with labels
cropped_faces = []
# Process each detected face
for (x, y, w, h) in faces:
face_count += 1
# Draw rectangles around the detected faces
cv2.rectangle(img, (x, y), (x + w, y + h), (255, 0, 0), 2)
# Extract the face region
face_img = img[y:y+h, x:x+w]
# Save the face image temporarily
face_img_path="temp_face.jpg"
cv2.imwrite(face_img_path, face_img)
# Detect gender using the InferenceHTTPClient
result = CLIENT.infer(face_img_path, model_id="gender-detection-qiyyg/2")
if 'predictions' in result and result['predictions']:
prediction = result['predictions'][0]
gender = prediction['class']
confidence = prediction['confidence']
# Label the rectangle with the gender and confidence
label = f'{gender} ({confidence:.2f})'
cv2.putText(img, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.8, (255, 0, 0), 2)
# Add the cropped face with label to the list
cropped_faces.append((face_img, label))
For each recognized face, the system draws a bounding box using: cv2.rectangle()
Visually highlight the face in the image. Then slice it (face_img = img[y:y+h, x:x+w]
), and isolates them for further processing. After temporarily storing the cropped faces, the system passes them through the Roboflow model. CLIENT.infer()
This returns a gender prediction along with a confidence score. The system adds these results as text labels above each face. cv2.putText()
Provides clear and informative overlays.
Step 6: Display Results
Finally, we visualize the output. First, we convert the image from BGR to RGB (OpenCV uses BGR by default). Then, we display the detected faces and their gender predictions. Then, we display each cropped face with its corresponding label.
# Convert image from BGR to RGB for display
img_rgb = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# Display the image with detected faces and gender labels
plt.figure(figsize=(10, 10))
plt.imshow(img_rgb)
plt.axis('off')
plt.title(f"Detected Faces: {face_count}")
plt.show()
# Display each cropped face with its label horizontally
fig, axes = plt.subplots(1, face_count, figsize=(15, 5))
for i, (face_img, label) in enumerate(cropped_faces):
face_rgb = cv2.cvtColor(face_img, cv2.COLOR_BGR2RGB)
axes[i].imshow(face_rgb)
axes[i].axis('off')
axes[i].set_title(label)
plt.show()
- Image conversion: Since OpenCV uses BGR format by default, we convert the image to RGB using cv2.cvtColor() for correct color display in Matplotlib.
- Show results:
- We use Matplotlib to display an image with the detected faces and their gender labels.
- We also show each cropped face image and its predicted gender label in separate subplots.
Original data
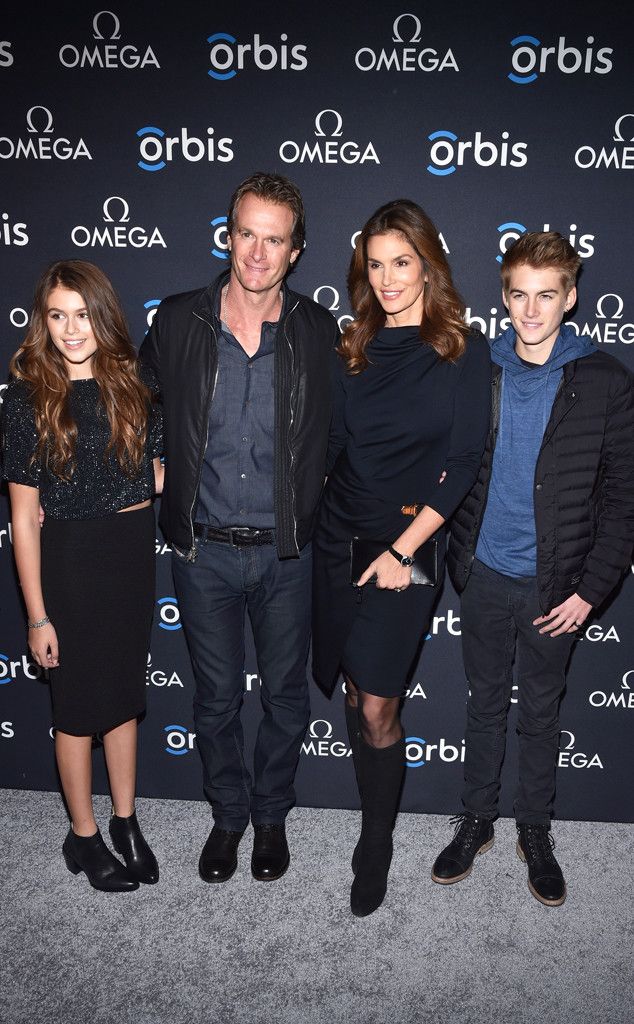
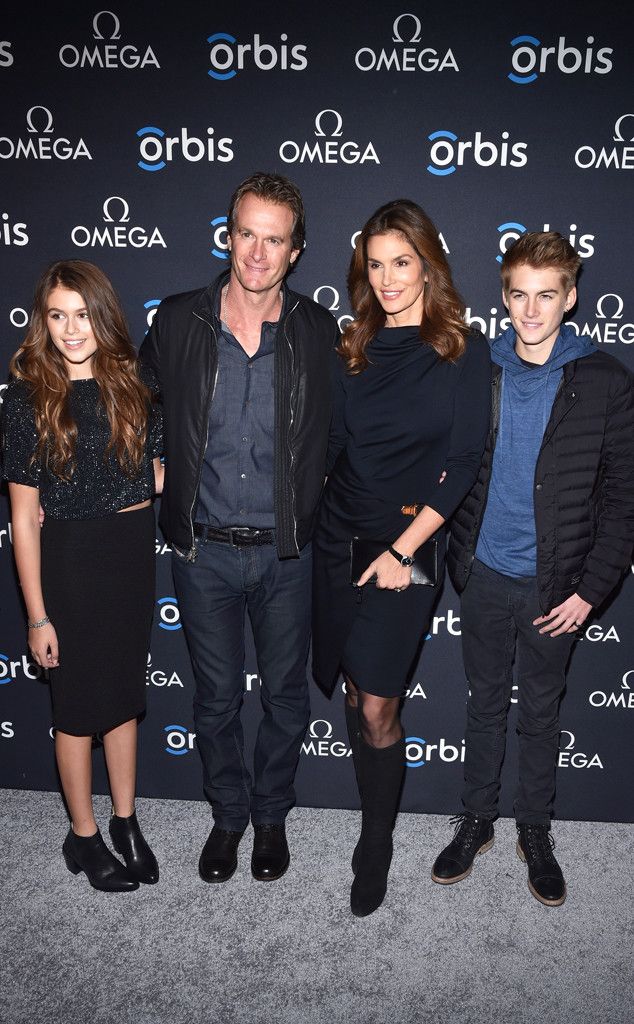
Output result data
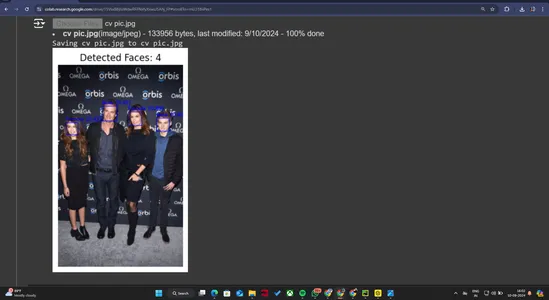
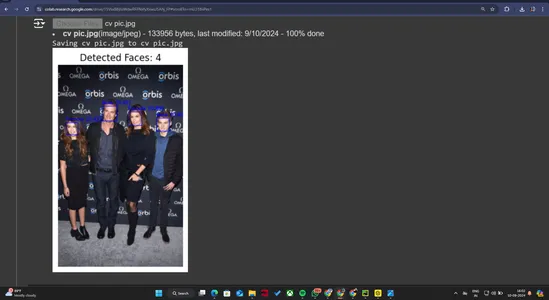
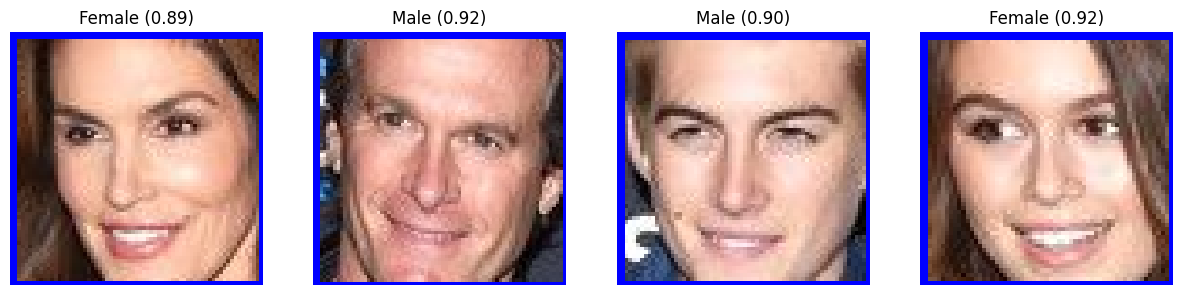
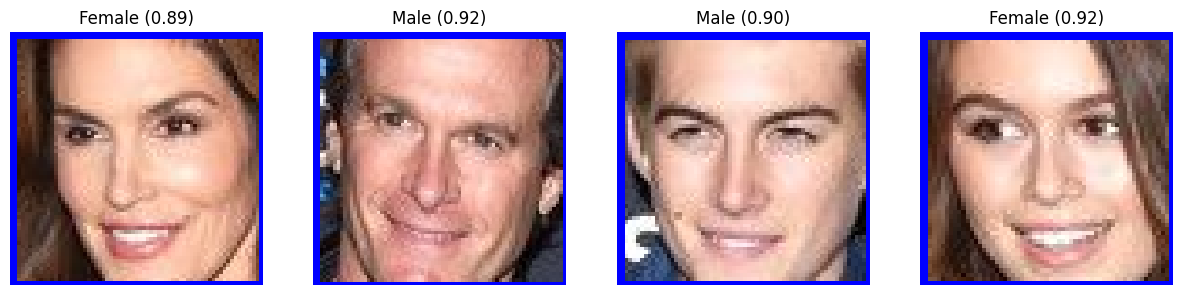
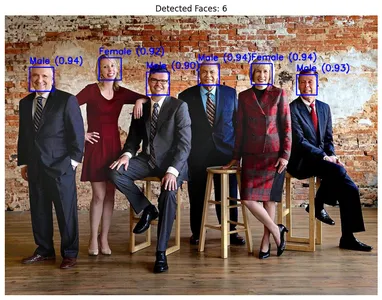
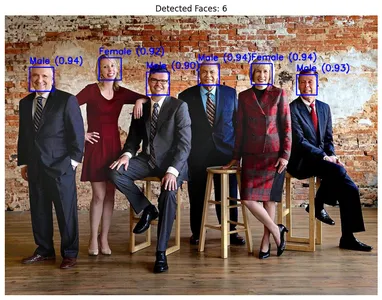
conclusion
In this tutorial, we successfully developed a robust gender detection using OpenCV and Roboflow in Python. By implementing OpenCV for face detection and Roboflow for gender prediction, we created a system that can accurately identify and classify gender in images. Adding Matplotlib for visualization further enhances the project, allowing us to present the results clearly and insightfully. This project highlights the effectiveness of combining these techniques, demonstrates practical benefits in real-world applications, and provides a powerful solution for gender detection tasks.
Main Content
- This project demonstrates an effective approach to detect and classify gender in images using pre-trained AI models. This demo demonstrates unwavering quality by accurately distinguishing sexual orientation with high certainty.
- The venture effectively combines various innovations to achieve its goal by combining devices such as Roboflow for AI inference, OpenCV for image preparation, and Matplotlib for visualization.
- This system has a remarkable ability to distinguish and classify the gender of different people in a single photo, making it suitable for a wide range of fields.
- Using pre-trained demos ensures the accuracy of predictions, which is evidenced by the certainty scores given below. This accuracy is essential for applications that require reliable gender classification.
- This project used visualization techniques to annotate images with detected faces and predicted genders, making the results more interpretable and valuable for further analysis.
Also Read: Name-Based Gender Identification Using NLP and Python
Frequently Asked Questions
A. This project aims to detect and classify gender in images using AI. It uses a pre-trained model to identify and label the gender of an individual in a photo.
A. This project used the Roboflow gender detection model for AI inference, OpenCV for image processing, and Matplotlib for visualization. Python was also used for scripting and data processing.
A. The model analyzes the image to detect faces, then classifies each detected face as male or female based on the trained AI algorithm. It outputs a confidence score for the prediction.
A. This model shows high accuracy, and the confidence score indicates a reliable prediction. For example, the confidence score of the result is over 80%, which shows strong performance.
Media displayed in this article are not owned by Analytics Vidhya and are used at the author’s discretion.